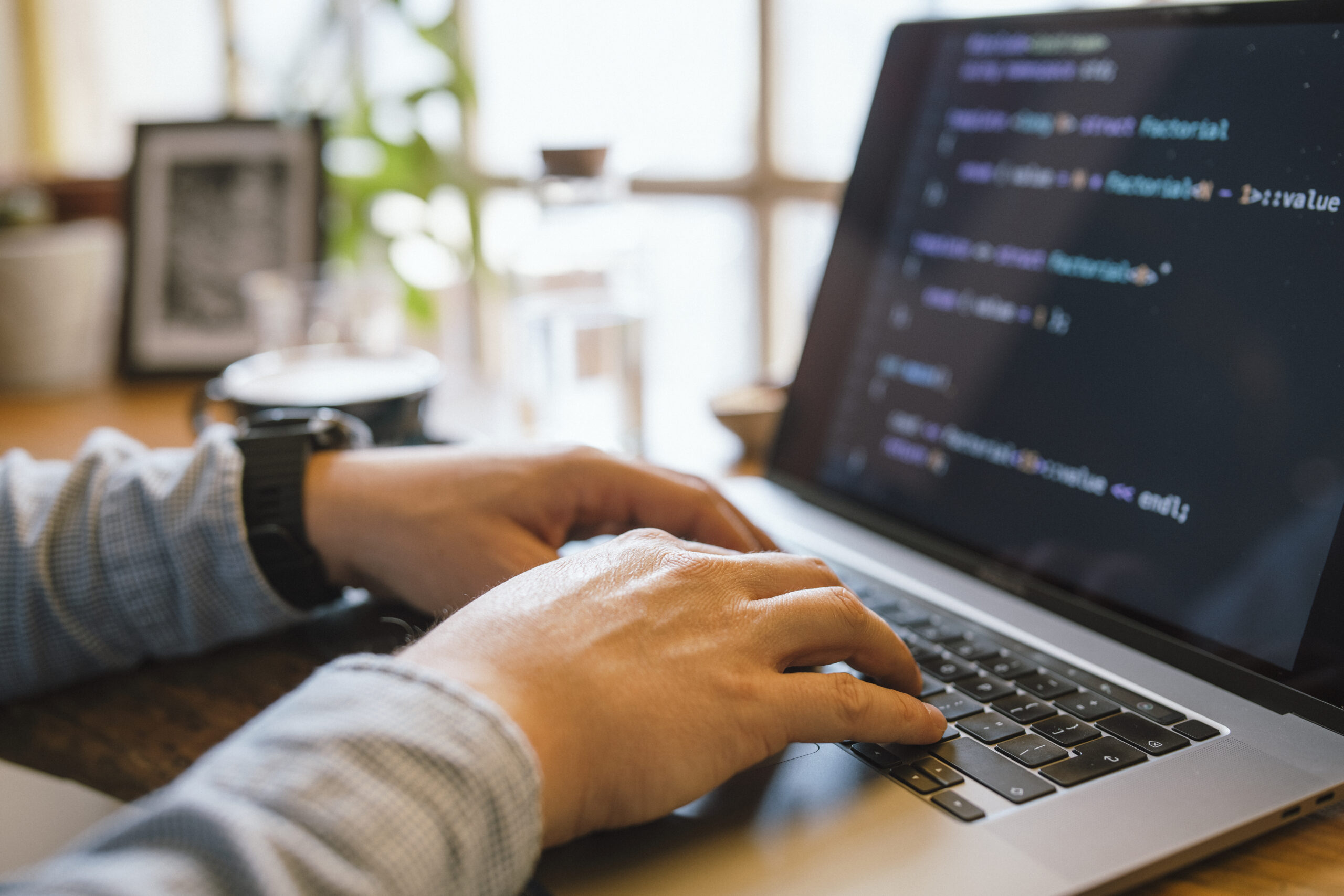
Debugging is one of the most vital — nonetheless frequently disregarded — techniques inside of a developer’s toolkit. It isn't nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and learning to think methodically to solve issues effectively. No matter whether you are a novice or possibly a seasoned developer, sharpening your debugging capabilities can help save hrs of stress and substantially increase your productiveness. Allow me to share many approaches to help you developers level up their debugging sport by me, Gustavo Woltmann.
Master Your Applications
On the list of fastest approaches developers can elevate their debugging capabilities is by mastering the tools they use every day. Even though writing code is one particular A part of progress, being aware of ways to communicate with it correctly throughout execution is equally vital. Modern advancement environments appear Geared up with impressive debugging capabilities — but numerous developers only scratch the surface of what these resources can do.
Consider, one example is, an Integrated Progress Ecosystem (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These instruments help you set breakpoints, inspect the worth of variables at runtime, step as a result of code line by line, as well as modify code on the fly. When used the right way, they Permit you to notice just how your code behaves in the course of execution, that is a must have for tracking down elusive bugs.
Browser developer tools, which include Chrome DevTools, are indispensable for front-end developers. They permit you to inspect the DOM, observe network requests, view serious-time functionality metrics, and debug JavaScript inside the browser. Mastering the console, resources, and community tabs can switch frustrating UI troubles into workable responsibilities.
For backend or procedure-level developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB offer deep Management around managing procedures and memory administration. Studying these instruments may have a steeper Finding out curve but pays off when debugging overall performance difficulties, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be snug with version Manage devices like Git to understand code historical past, come across the precise moment bugs were introduced, and isolate problematic alterations.
In the long run, mastering your tools indicates heading over and above default options and shortcuts — it’s about producing an personal understanding of your advancement setting to make sure that when challenges crop up, you’re not missing in the dead of night. The higher you already know your instruments, the more time you are able to invest resolving the particular difficulty instead of fumbling as a result of the procedure.
Reproduce the challenge
Probably the most significant — and sometimes missed — methods in effective debugging is reproducing the issue. In advance of jumping in the code or creating guesses, builders require to produce a steady environment or situation where the bug reliably seems. Without reproducibility, repairing a bug becomes a match of prospect, frequently leading to wasted time and fragile code variations.
The initial step in reproducing a problem is gathering as much context as you possibly can. Request issues like: What steps led to The difficulty? Which environment was it in — improvement, staging, or manufacturing? Are there any logs, screenshots, or error messages? The greater element you might have, the less complicated it will become to isolate the exact circumstances under which the bug takes place.
As soon as you’ve gathered sufficient facts, make an effort to recreate the problem in your local atmosphere. This may necessarily mean inputting the same data, simulating identical consumer interactions, or mimicking method states. If the issue seems intermittently, contemplate creating automatic exams that replicate the sting situations or point out transitions concerned. These checks not simply assist expose the condition but in addition prevent regressions Sooner or later.
Sometimes, The problem may be setting-specific — it would materialize only on particular running units, browsers, or under certain configurations. Working with tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms might be instrumental in replicating these types of bugs.
Reproducing the trouble isn’t merely a move — it’s a attitude. It calls for endurance, observation, and also a methodical solution. But once you can regularly recreate the bug, you're currently midway to correcting it. That has a reproducible circumstance, you can use your debugging applications additional proficiently, test prospective fixes securely, and communicate additional Plainly using your staff or end users. It turns an abstract criticism into a concrete challenge — and that’s in which developers prosper.
Study and Understand the Error Messages
Error messages in many cases are the most worthy clues a developer has when one thing goes wrong. In lieu of seeing them as frustrating interruptions, developers should understand to treat error messages as immediate communications from your system. They usually show you precisely what occurred, where it took place, and in some cases even why it happened — if you understand how to interpret them.
Start out by studying the message thoroughly As well as in full. Several developers, particularly when underneath time force, glance at the primary line and promptly get started making assumptions. But further in the mistake stack or logs may perhaps lie the true root result in. Don’t just copy and paste error messages into search engines like google — read through and recognize them initially.
Break the error down into pieces. Could it be a syntax error, a runtime exception, or maybe a logic error? Will it issue to a certain file and line range? What module or functionality induced it? These concerns can guideline your investigation and place you toward the responsible code.
It’s also handy to grasp the terminology of the programming language or framework you’re applying. Mistake messages in languages like Python, JavaScript, or Java usually follow predictable designs, and learning to acknowledge these can dramatically increase your debugging approach.
Some errors are obscure or generic, As well as in Individuals conditions, it’s vital to look at the context wherein the mistake occurred. Verify connected log entries, input values, and recent changes within the codebase.
Don’t overlook compiler or linter warnings possibly. These generally precede much larger troubles and provide hints about possible bugs.
Finally, mistake messages usually are not your enemies—they’re your guides. Understanding to interpret them properly turns chaos into clarity, encouraging you pinpoint challenges more rapidly, lower debugging time, and turn into a additional economical and confident developer.
Use Logging Properly
Logging is Among the most powerful resources within a developer’s debugging toolkit. When applied correctly, it offers true-time insights into how an application behaves, serving to you fully grasp what’s going on beneath the hood without needing to pause execution or step from the code line by line.
A great logging technique starts off with figuring out what to log and at what degree. Frequent logging levels involve DEBUG, Data, Alert, Mistake, and FATAL. Use DEBUG for specific diagnostic data in the course of enhancement, Data for common situations (like profitable begin-ups), Alert for possible difficulties that don’t break the application, Mistake for actual troubles, and Lethal once the process can’t continue.
Keep away from flooding your logs with too much or irrelevant information. Excessive logging can obscure important messages and slow down your process. Focus on crucial functions, point out changes, input/output values, and critical selection factors with your code.
Structure your log messages Obviously and persistently. Involve context, such as timestamps, ask for IDs, and performance names, so it’s simpler to trace concerns in dispersed devices or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even simpler to parse and filter logs programmatically.
Through debugging, logs Enable you to monitor how variables evolve, what circumstances are met, and what branches of logic are executed—all without halting This system. They’re In particular worthwhile in production environments wherever stepping by way of code isn’t achievable.
In addition, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that support log rotation, filtering, and integration with checking dashboards.
In the end, wise logging is about harmony and clarity. Using a well-considered-out logging strategy, you can decrease the time it takes to identify concerns, gain deeper visibility into your purposes, and Enhance the In general maintainability and dependability of your code.
Consider Similar to a Detective
Debugging is not only a complex task—it's a sort of investigation. To effectively discover and take care of bugs, builders must method the process just like a detective fixing a thriller. This mindset aids break down advanced challenges into workable parts and adhere to clues logically to uncover the root click here result in.
Start off by collecting evidence. Look at the indicators of the challenge: error messages, incorrect output, or functionality troubles. Similar to a detective surveys a criminal offense scene, gather as much relevant information as you are able to with out leaping to conclusions. Use logs, examination situations, and user reports to piece alongside one another a clear picture of what’s going on.
Up coming, variety hypotheses. Question on your own: What can be producing this habits? Have any improvements just lately been created for the codebase? Has this concern occurred before less than very similar situation? The purpose is always to narrow down choices and identify prospective culprits.
Then, check your theories systematically. Try and recreate the trouble inside a managed setting. Should you suspect a particular function or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, question your code concerns and Enable the outcome lead you nearer to the truth.
Pay shut focus to small facts. Bugs typically hide from the least envisioned areas—similar to a missing semicolon, an off-by-a person mistake, or perhaps a race affliction. Be comprehensive and affected individual, resisting the urge to patch The problem without the need of completely understanding it. Momentary fixes might cover the real dilemma, just for it to resurface later on.
And lastly, maintain notes on what you tried out and discovered. Equally as detectives log their investigations, documenting your debugging procedure can help save time for future troubles and help Other folks understand your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical techniques, approach troubles methodically, and come to be more effective at uncovering hidden troubles in elaborate methods.
Produce Checks
Creating assessments is among the most effective strategies to help your debugging expertise and In general improvement efficiency. Exams not simply enable capture bugs early but also serve as a safety Internet that provides you self-assurance when building variations to your codebase. A nicely-tested application is simpler to debug as it means that you can pinpoint accurately where by and when a problem occurs.
Start with unit tests, which give attention to specific features or modules. These modest, isolated assessments can promptly expose irrespective of whether a selected bit of logic is Doing the job as predicted. Every time a take a look at fails, you promptly know where by to glance, appreciably lessening enough time put in debugging. Unit tests are especially useful for catching regression bugs—challenges that reappear just after Earlier currently being mounted.
Subsequent, integrate integration tests and close-to-conclusion exams into your workflow. These assist make sure that various aspects of your application function alongside one another efficiently. They’re specifically helpful for catching bugs that manifest in advanced techniques with multiple parts or providers interacting. If some thing breaks, your checks can inform you which A part of the pipeline unsuccessful and beneath what conditions.
Producing exams also forces you to Assume critically about your code. To check a attribute correctly, you need to be aware of its inputs, anticipated outputs, and edge conditions. This amount of comprehending naturally sales opportunities to better code framework and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug can be a strong starting point. After the take a look at fails regularly, you may concentrate on repairing the bug and watch your examination go when the issue is settled. This solution ensures that precisely the same bug doesn’t return Down the road.
In brief, composing checks turns debugging from a disheartening guessing recreation into a structured and predictable system—aiding you capture extra bugs, quicker and a lot more reliably.
Choose Breaks
When debugging a tricky problem, it’s effortless to be immersed in the condition—staring at your display for hours, attempting Resolution immediately after solution. But Probably the most underrated debugging resources is just stepping away. Getting breaks can help you reset your intellect, decrease disappointment, and sometimes see The problem from the new point of view.
When you are way too near to the code for far too very long, cognitive exhaustion sets in. You may perhaps start overlooking obvious errors or misreading code that you simply wrote just hours earlier. Within this state, your brain gets to be much less efficient at problem-resolving. A brief stroll, a coffee break, or even switching to a different task for ten–quarter-hour can refresh your concentration. Quite a few builders report locating the root of a problem when they've taken time and energy to disconnect, allowing their subconscious function within the background.
Breaks also assistance protect against burnout, Specially in the course of lengthier debugging classes. Sitting down in front of a monitor, mentally caught, is not merely unproductive but also draining. Stepping absent lets you return with renewed Vitality along with a clearer mentality. You would possibly abruptly notice a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you before.
When you’re stuck, a very good guideline is to established a timer—debug actively for forty five–60 minutes, then have a 5–ten minute split. Use that time to maneuver around, extend, or do something unrelated to code. It could really feel counterintuitive, Primarily below limited deadlines, nevertheless it actually brings about quicker and more practical debugging In the end.
Briefly, taking breaks is just not an indication of weakness—it’s a wise system. It provides your Mind House to breathe, improves your point of view, and helps you stay away from the tunnel eyesight That always blocks your progress. Debugging can be a psychological puzzle, and rest is a component of fixing it.
Master From Each and every Bug
Just about every bug you encounter is much more than simply A short lived setback—it's a chance to increase to be a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each can train you a little something valuable should you make the effort to replicate and analyze what went Improper.
Start off by inquiring on your own a handful of key concerns after the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved procedures like unit screening, code testimonials, or logging? The solutions typically reveal blind spots within your workflow or comprehension and make it easier to Make more robust coding behaviors transferring ahead.
Documenting bugs can even be a fantastic routine. Hold a developer journal or keep a log where you Be aware down bugs you’ve encountered, how you solved them, and what you learned. Over time, you’ll begin to see designs—recurring concerns or frequent blunders—which you could proactively keep away from.
In crew environments, sharing Everything you've discovered from the bug with all your friends could be Particularly powerful. Whether it’s via a Slack concept, a short generate-up, or A fast information-sharing session, assisting Many others stay away from the identical issue boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. In place of dreading bugs, you’ll begin appreciating them as essential portions of your advancement journey. After all, many of the very best builders usually are not those who compose fantastic code, but individuals who continuously understand from their mistakes.
In the long run, each bug you correct provides a fresh layer towards your ability established. So subsequent time you squash a bug, have a moment to mirror—you’ll occur away a smarter, far more able developer as a result of it.
Summary
Improving your debugging capabilities takes time, apply, and endurance — but the payoff is big. It would make you a more effective, self-confident, and able developer. The next time you are knee-deep in a very mysterious bug, remember: debugging isn’t a chore — it’s a chance to be improved at what you do.